Schema validation for Node.js with help of AI
TypeScript interfaces and Zod validation schemas are both valuable tools for developers to ensure type safety and prevent errors in their code. In this article, I will demonstrate how to use TypeScript interfaces with the help of ChatGPT to define Zod validation schemas.
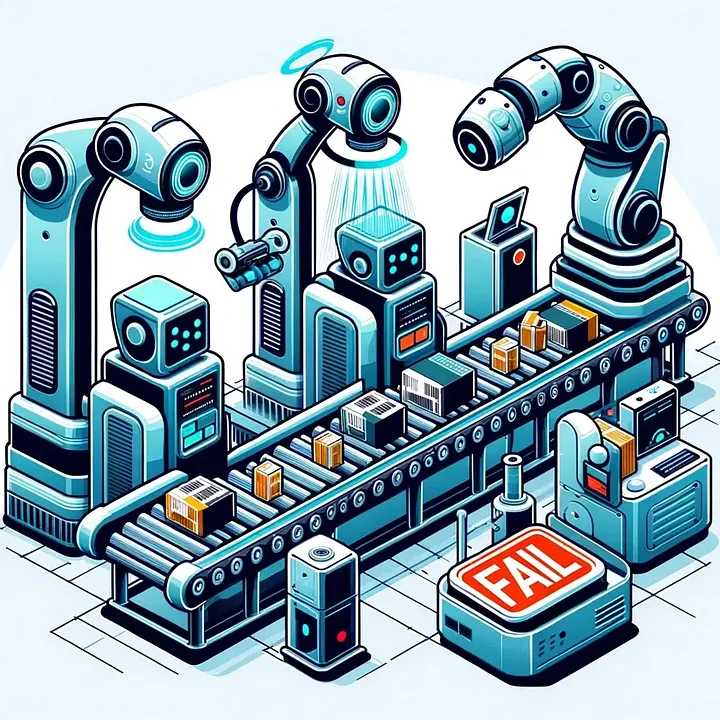
TypeScript Interfaces and Zod Validation Schemas: A Powerful Combination for Type Safety.
Introduction to Zod
Zod is an easy-to-use and declarative JavaScript validation library that helps verify variables and objects based on TypeScript types. With Zod, you can specify the data you expect from an object and check those types at runtime.
Using TypeScript Interfaces
First, let’s define a simple TypeScript interface for an example object:
interface User {
id: number;
name: string;
email: string;
}
This User interface contains type information related to a user. With this interface in place, we can use it to create a Zod validation schema.
Creating a Zod Validation Schema
With Zod, you can easily create a validation schema based on the TypeScript interface. Here’s how you can do it:
import { z } from "zod";
const userSchema = z.object({
id: z.number(),
name: z.string(),
email: z.string().email(),
});
This code creates a Zod schema named userSchema that corresponds to the type defined by the User interface. You can also add Zod rules, such as email validation, as shown in the example.
Checking Objects with Zod
Now that we have the userSchema, we can use it to check objects. For example, if you receive a user object from somewhere, like a form, you can validate it against the schema:
const userData = {
id: 1,
name: "John Doe",
email: "johndoe@example.com",
};
try {
const validatedUser = userSchema.parse(userData);
console.log("User data is valid:", validatedUser);
} catch (error) {
console.error("Invalid user data:", error);
}
In this example, we validate the userData object according to the userSchema. If the data matches the schema, the validatedUser variable contains the validated data. If there are errors in the data, the parse method throws an exception that can be handled.
Enlisting AI’s Help
The above example illustrates a straightforward case where creating a validation schema based on an interface is not a significant effort. But what should you do when you need to handle nested objects or when you have to write validation for too many fields?
In such cases, the ChatGPT or even GitHub co-pilot can provide excellent assistance. You can use the following prompt if you already have TypeScript interfaces:
interface User {
id: number;
name: string;
email: string;
address?: string;
cars: Car[];
}
interface Car {
type: string;
plateNumber: string;
age: number;
color?: string;
}
The output of this prompt will be something like this:
import { z } from "zod";
// Define the Car schema
const CarSchema = z.object({
type: z.string(),
plateNumber: z.string(),
age: z.number(),
color: z.string().optional(),
});
// Define the User schema using the Car schema
const UserSchema = z.object({
id: z.number(),
name: z.string(),
email: z.string(),
address: z.string().optional(),
cars: z.array(CarSchema),
});
// Example usage:
const user = {
id: 1,
name: "John Doe",
email: "john@example.com",
address: "123 Main St",
cars: [
{
type: "Sedan",
plateNumber: "ABC123",
age: 3,
color: "Red",
},
// Include other Car objects as needed
],
};
try {
const validatedUser = UserSchema.parse(user);
console.log(validatedUser);
} catch (error) {
console.error(error.message);
}
You can now use this code in your project, eliminating the need to manually write and test the schema.
Conclusion
Using TypeScript interfaces with Zod validation schemas can easily provide type safety and error handling in TypeScript projects. These schemas help prevent runtime errors and improve code quality. Fortunately, this can now be generated easily with the help of AI, ensuring consistent behavior. The combination of AI, Zod, and TypeScript is an excellent tool for developing reliable and stable applications.